Published
- 6 min read
CJS and ESM Harmony: Magic of Rollup & Terser
Key Takeaways
- ✨ Optimize your TypeScript package with easy configuration for CJS and ESM compatibility.
- ✨ Rollup, a module bundler that effectively compiles and bundles your code.
- ✨ Terser, a TypeScript parser and compressor, to minify code and reduce bundle size.
- ✨ Achieve a 58% reduction in package size by leveraging Rollup and Terser for bundling and minification.
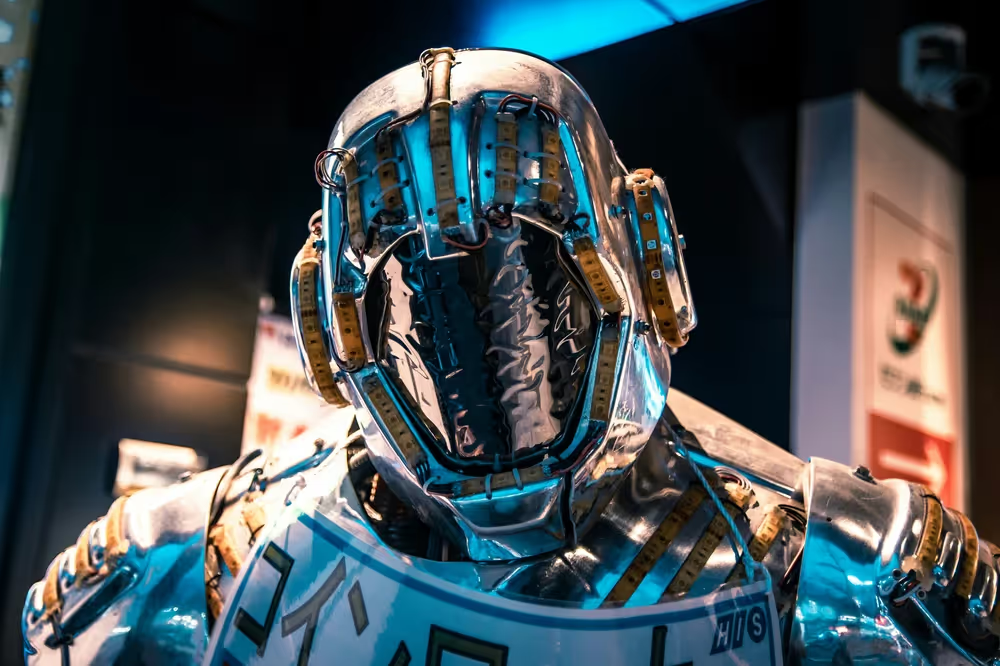
Photo by Maximalfocus on Unsplash
In the fast-paced world of software development, efficiency and performance are paramount. As developers, we constantly seek ways to optimize our code, reduce package sizes, and enhance load times. However, one of the most challenging aspects of modern JavaScript development is ensuring compatibility with both CommonJS (CJS) and ES Modules (ESM). Many libraries and applications need to support both module systems, which often requires complex configurations and build processes.
The Challenge 🌟
When I first developed Hashtegrity, a powerful library designed for generating hashes and ensuring data integrity, I was delighted with its capabilities. However, as the library expanded, so did its size, reaching a hefty 353KB. This was becoming a burden, particularly for users seeking a lightweight solution for their projects.
I realized the need to optimize the package without sacrificing its robust features. This led me to explore various bundling and minification tools. After extensive research and experimentation, I discovered the ideal combination: Rollup and Terser. This duo not only addressed the complexities of CJS and ESM compatibility but also achieved a significant 58% reduction in package size, enhancing both performance and usability. 🎯
Enter Rollup: The Magic Bundler 🪄
Rollup is a powerful module bundler for JavaScript, designed to compile small pieces of code into larger, more complex structures like libraries or applications. What sets Rollup apart from other bundlers is its exceptional tree-shaking capability, which effectively removes unused code, thereby reducing the overall bundle size.
Setting Up Rollup 🔧
To begin using Rollup, I first installed the necessary dependencies.
npm install rollup @rollup/plugin-commonjs @rollup/plugin-node-resolve @rollup/plugin-typescript @rollup/plugin-terser --save-dev
Next, I created a rollup.config.js
file to configure Rollup in the root directory:
import commonjs from '@rollup/plugin-commonjs'import resolve from '@rollup/plugin-node-resolve'import typescript from '@rollup/plugin-typescript'import { terser } from '@rollup/plugin-terser'
const external = [...Object.keys(require('./package.json').dependencies || {})]
export default { input: 'src/index.ts', output: [ { file: 'dist/index.cjs', format: 'cjs' }, { file: 'dist/index.mjs', format: 'esm' } ], plugins: [ resolve(), commonjs(), typescript({ tsconfig: './tsconfig.json' }), terser() ], external}
In this configuration, I specified the input file as src/index.ts
and defined the output files as index.cjs
for CommonJS and index.mjs
for ES Modules. The formats cjs
and esm
were chosen to ensure compatibility with both module systems. Additionally, I included essential plugins for module resolution, handling CommonJS modules, compiling TypeScript, and minifying the code with Terser.
Building the Package 🏗️
With the configuration set, I added a build script to my package.json
:
"scripts": { "build": "rollup -c"}
Running the build script was straightforward and involved a simple command:
npm run build
This setup allowed me to efficiently bundle my TypeScript code, addressing the challenges of CJS and ESM compatibility while significantly reducing the package size.
The Power of Terser: Minification ⚡
While Rollup did an excellent job of bundling the code, I needed something more to achieve the desired reduction in size. That’s where Terser came into play. Terser is a JavaScript parser and mangler/compressor toolkit for ES6+.
Integrating Terser 🔍
Integrating Terser with Rollup was straightforward, thanks to the Terser plugin already included in my Rollup configuration. This plugin minifies the bundled code by removing unnecessary whitespace, comments, and other redundant elements. The minification process significantly reduces the bundle size without compromising the code’s functionality.
import { terser } from '@rollup/plugin-terser'
export default { // ... other configurations plugins: [ // ... other plugins terser() ]}
By leveraging Terser’s capabilities, I was able to further optimize the package, ensuring that the final output was as compact and efficient as possible. This step was crucial in achieving the impressive 58% reduction in package size, enhancing both the performance and load times of the library.
Enhancing Developer Experience 🚀
To provide a seamless developer experience and ensure that your library is easy to use, it’s important to include TypeScript declaration files in your package. These files offer type definitions that help developers understand how to interact with your library effectively.
To achieve this, update your tsconfig.json
file with the following settings:
{ "compilerOptions": { // Other Compiler options... "declaration": true, "declarationMap": true, "declarationDir": "./dist/types" } // Other tsconfig options...}
These settings ensure that TypeScript generates declaration files (.d.ts
) and places them in the specified types
directory. By including these files in your package, you enhance the usability of your library, making it easier for developers to integrate and work with your code confidently.
Configuring the Exports Section 📦
To ensure that your package is compatible with both ES Modules (ESM) and CommonJS (CJS), it’s essential to define an exports
section in your package.json
file. This section specifies the paths to the ESM and CJS bundles, allowing users to import your package using either module syntax.
Here’s how you can configure the exports
section:
{ "main": "dist/index.cjs", "module": "dist/index.mjs", "types": "dist/types/index.d.ts", "type": "module", "exports": { ".": { "import": "./dist/index.mjs", "require": "./dist/index.cjs", "types": "./dist/types/index.d.ts" } }}
This configuration directs Node.js to use the appropriate module format based on the import method. By clearly defining these paths, you enhance the versatility of your package, making it easier to integrate into various projects and ensuring seamless compatibility across different environments.
Tips for Optimization 💡
- Tree-Shaking: Ensure your code is modular and uses ES6 import/export syntax to fully leverage Rollup’s tree-shaking capabilities, which help eliminate unused code and reduce bundle size.
- Code Splitting: For larger projects, consider using Rollup’s code-splitting feature to create smaller, more manageable bundles. This can improve load times and make your application more efficient.
- Source Maps: Enable source maps in your Rollup configuration to facilitate easier debugging. This allows you to trace back minified code to its original source, making it simpler to identify and fix issues.
Wrapping Up: The Rollup and Terser Adventure 🎉
Embarking on the journey to optimize Hashtegrity with Rollup and Terser was like setting sail on a thrilling adventure. By trimming the package size from a hefty 353KB to a sleek 149KB, I transformed the library into a nimble powerhouse. Rollup’s magic made it a breeze to juggle both ESM and CJS, while Terser swooped in to give the final polish. This dynamic duo not only boosted performance but also made the library a joy to use across different environments.
I hope this tale of transformation inspires you to unleash the power of Rollup and Terser in your own projects. So, grab your coding gear and set off on your own optimization quest.
Happy coding, and may your bundles be ever lean! 🎉
Advertisement